Understanding the various types of constructors is pivotal for developers to craft efficient and organized code. These constructors cater to different needs, allowing for object initialization with default values, parameters, and even facilitating unique scenarios such as overloading, copying, or ensuring the singleton pattern. Exploring the nuances of each constructor type enables programmers to wield greater control over object creation, setting the stage for robust and effective application development in C#.
- Introduction to Constructors in C#
- Default Constructor
- Parameterized Constructor
- Constructor Overloading
- Copy Constructor
- Static Constructor
- Private Constructor
- Difference between Constructors and Methods
- Best Practices for Using Constructors
- Constructor Chaining
- Initialization in Constructors
- Constructor Accessibility Modifiers
- Real-life Examples of Constructor Usage
- Common Errors and Pitfalls with Constructors
- Conclusion
Constructors in C# are fundamental elements that facilitate the creation and initialization of objects within a class. They are special methods responsible for initializing objects and allocating memory. Understanding the various types of constructors in C# is crucial for efficient programming and proper object initialization.
Types of Constructors
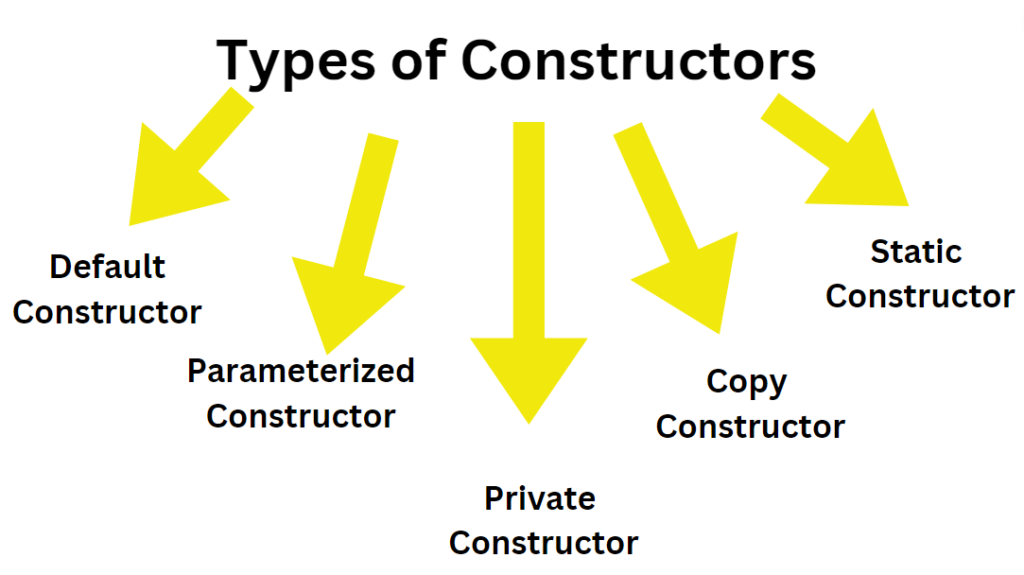
1. Default Constructor
The default constructor in C# is automatically generated by the compiler if no constructor is explicitly defined within a class. It initializes the object’s variables to their default values. For instance, in C#, a default constructor can be defined as:
public class MyClass
{
// Default Constructor
public MyClass()
{
// Initialization code
}
}
2. Parameterized Constructor
Contrary to the default constructor, a parameterized constructor allows developers to pass parameters during object instantiation. It enables the initialization of object properties with specific values based on the provided arguments.
public class Employee
{
public string Name;
public int Age;
// Parameterized Constructor
public Employee(string name, int age)
{
Name = name;
Age = age;
}
}
3. Constructor Overloading
Constructor overloading involves creating multiple constructors within the same class with different parameter lists. It allows for flexibility when initializing objects, catering to various initialization scenarios.
4. Copy Constructor
In C#, a copy constructor is used to create a new object as a copy of an existing object. It copies the values of the properties from one object to another.
5. Static Constructor
Static constructors are used to initialize static data members of a class. They are called only once, ensuring that static members are initialized before the first object of the class is created or any static member is accessed.
6. Private Constructor
A private constructor restricts the instantiation of objects from outside the class. It is commonly used in singleton design patterns, ensuring only one instance of a class can be created.
7. Difference between Constructors and Methods
Constructors are distinct from methods in C#. They have no return type and are automatically called upon object creation, primarily for initializing object state, while methods perform specific actions and can be invoked multiple times.
8. Best Practices for Using Constructors
When utilizing constructors, it’s recommended to keep them concise, initialize variables, avoid complex logic, and follow naming conventions for clarity.
9. Constructor Chaining
Constructor chaining enables one constructor to call another constructor within the same class. This mechanism helps in reducing code duplication and ensuring proper initialization.
10. Initialization in Constructors
Constructors allow initialization of fields, properties, and other objects, ensuring the object starts in a valid state.
11. Constructor Accessibility Modifiers
Access modifiers like public, private, protected, and internal can be applied to constructors, controlling their accessibility from outside the class.
12. Real-life Examples of Constructor Usage
Explore practical instances where various types of constructors are employed in real-world scenarios, enhancing code clarity and efficiency.
13. Common Errors and Pitfalls with Constructors
Understanding common mistakes and pitfalls while working with constructors can aid in writing robust, error-free code.
14. Conclusion
Constructors in C# play a pivotal role in object initialization, providing flexibility and control over the creation of objects. By utilizing different types of constructors, developers can efficiently manage object state and behavior.
Please refer below book for more details:
15. FAQs
Q1: Can constructors be inherited in C#? A: Constructors are not inherited in the traditional sense in C#. However, constructors from a base class can be accessed using the base
keyword in derived classes, aiding in object initialization.
Q2: Is it possible to have a private static constructor in C#? A: Yes, a class can contain a private static constructor. This constructor is invoked only once to initialize static members of the class and cannot be directly called from outside the class.
Q3: Can a constructor be overloaded with different access modifiers in C#? A: Yes, constructors can be overloaded with varying access modifiers such as public, private, protected, or internal, allowing developers to control the accessibility of constructors within or outside the class.
Q4: What happens if a class in C# has no constructor defined explicitly? A: If no constructor is explicitly defined in a class, C# provides a default constructor by default. This default constructor initializes fields to their default values, aiding in object instantiation.
Q5: Can constructors in C# return values? A: Constructors do not return values. They are responsible for initializing objects and have no return type, serving the purpose of setting up an object’s initial state upon creation.
Q6: Can constructors be invoked explicitly in C#? A: Constructors are automatically invoked upon object creation. While they cannot be called explicitly, constructor chaining allows one constructor to call another within the same class using the this
keyword.
Q7: Is it possible to have multiple constructors in a C# class? A: Yes, C# supports constructor overloading, enabling the creation of multiple constructors within the same class with different parameter lists. This flexibility aids in catering to diverse object initialization requirements.
Q8: Can constructors have access modifiers in C#? A: Yes, constructors can have access modifiers like public, private, protected, or internal. These modifiers control the visibility and accessibility of the constructor within or outside the class.
Q9: What is the role of constructors in memory allocation in C#? A: Constructors play a vital role in allocating memory for objects in C#. They initialize the object’s state, allocate memory, and set up default values for the object’s fields and properties.
Q10: Are constructors used solely for initializing variables in C#? A: While initializing variables is one of their primary roles, constructors in C# are responsible for setting up the initial state of an object, which may involve variable initialization, object creation, and additional setup required for the object to function correctly.