Abstract class and interfaces are fundamental concepts in object-oriented programming (OOP). An abstract class provides a partial implementation for subclasses, while an interface defines a contract for classes to implement methods and properties. Both facilitate code reusability and promote abstraction in C#.
Table of Content
- Introduction to Abstract Class and Interface
- Purpose and Usage of Abstract Classes
- Purpose and Usage of Interfaces
- Syntax and Implementation Differences
- Inheritance and Multiple Implementations
- Access Modifiers and Members
- Flexibility and Extensibility
- When to Use Abstract Classes
- When to Use Interfaces
- Similarities and Differences Summarized
- Examples Demonstrating Abstract Classes
- Examples Demonstrating Interfaces
- Pros and Cons of Abstract Classes
- Pros and Cons of Interfaces
- Conclusion
Difference Between Abstract Class and Interface
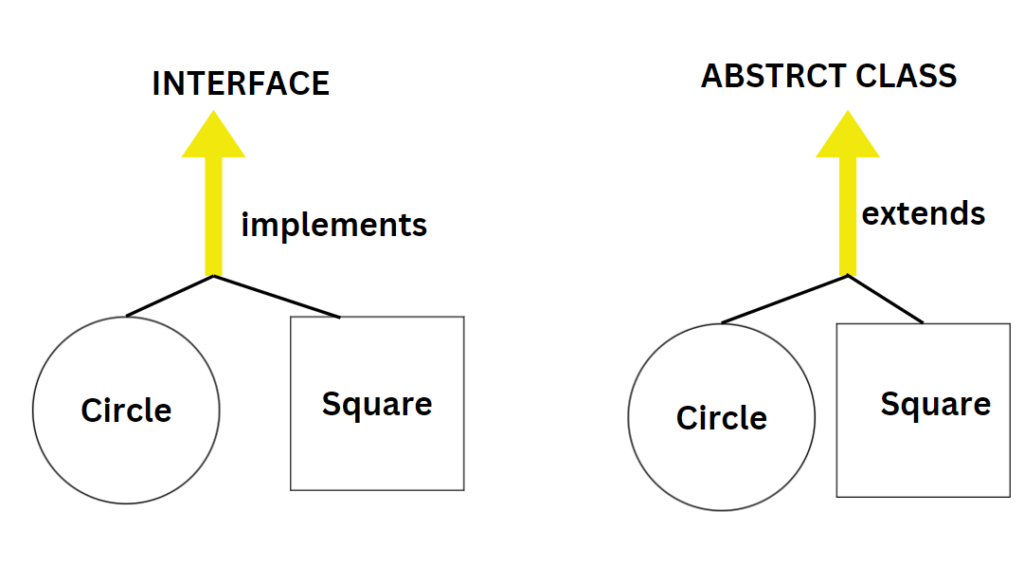
1. Introduction to Abstract Class and Interface
Abstract classes and interfaces are essential concepts in object-oriented programming. Both provide structures for defining methods and properties that can be inherited by classes. However, they have distinct features and purposes, influencing how they are utilized in C#.
2. Purpose and Usage of Abstract Classes
Abstract classes serve as blueprints for other classes to inherit from. They can contain abstract methods (methods without implementations) and concrete methods. Abstract classes cannot be instantiated but are meant to be extended by subclasses, which can then implement abstract methods.
3. Purpose and Usage of Interfaces
Interfaces, on the other hand, define a contract that classes can adhere to by implementing the interface’s methods and properties. They contain only method signatures, and a class can implement multiple interfaces, providing flexibility in defining behavior.
4. Syntax and Implementation Differences
Abstract classes use the abstract
keyword for method declaration, while interfaces use the interface
keyword to declare methods and properties. Abstract classes can have constructors, fields, and access modifiers, while interfaces can’t contain fields or implementation details.
5. Inheritance and Multiple Implementations
A class can inherit only one abstract class but implement multiple interfaces, enabling better flexibility in scenarios where multiple behaviors need to be incorporated.
6. Access Modifiers and Members
Abstract classes can have different access modifiers for their members, allowing more control over member visibility. Interfaces, however, have public access by default for their members.
7. Flexibility and Extensibility
Interfaces offer more flexibility in designing contracts as they can be implemented by various unrelated classes, promoting loose coupling and easy extensibility. Abstract classes, being more rigid due to single inheritance, provide a structured base for related subclasses.
8. When to Use Abstract Classes
Abstract classes are preferred when creating a blueprint with common methods for closely related classes where code reusability is a priority.
9. When to Use Interfaces
Interfaces are suitable for scenarios where multiple unrelated classes need to adhere to a specific contract or when a class requires multiple behaviors.
10. Similarities and Differences Summarized
While both abstract classes and interfaces facilitate abstraction and reusability, their differences lie in their implementation, inheritance, and usage scenarios.
11. Examples Demonstrating Abstract Classes
Consider a scenario where an abstract class Shape
defines an abstract method CalculateArea()
. Subclasses like Circle
and Rectangle
extend Shape
and implement CalculateArea()
with their specific formulas.
12. Examples Demonstrating Interfaces
An interface IResizable
may include methods like ResizeWidth()
and ResizeHeight()
. Classes like Window
and Image
implementing IResizable
will implement these methods based on their specific requirements.
13. Pros and Cons of Abstract Classes
Abstract classes offer better flexibility with method implementations and can include shared functionality. However, they limit subclassing due to single inheritance.
14. Pros and Cons of Interfaces
Interfaces promote loose coupling, allowing diverse classes to adhere to a common contract. Yet, implementing interfaces may lead to code duplication for shared method signatures.
15. Conclusion
In summary, both abstract classes and interfaces play crucial roles in C#, offering distinct advantages based on the design requirements. Understanding their differences enables developers to choose the appropriate abstraction mechanism for efficient code design.
Please refer below book for more details:
FAQs
Q1: Can a class inherit from multiple abstract classes in C#?
A: No, C# does not support multiple inheritance with abstract classes. A class can inherit from only one abstract class.
Q2: Can an abstract class implement interfaces in C#?
A: Yes, an abstract class in C# can implement interfaces, allowing it to define common behavior while providing a structured base for subclasses.
Q3: Is it possible to create an object of an abstract class in C#?
A: No, abstract classes cannot be instantiated directly. They serve as blueprints for subclasses and must be extended to create objects.
Q4: How do interfaces promote loosely coupled code in C#?
A: Interfaces define contracts that classes can adhere to without being tightly bound to each other’s implementations, promoting flexibility and ease of extension.
Q5: What happens if a class doesn’t implement all methods of an interface in C#?
A: In C#, if a class claims to implement an interface but doesn’t implement all its methods, it will result in a compilation error, ensuring adherence to the interface contract.