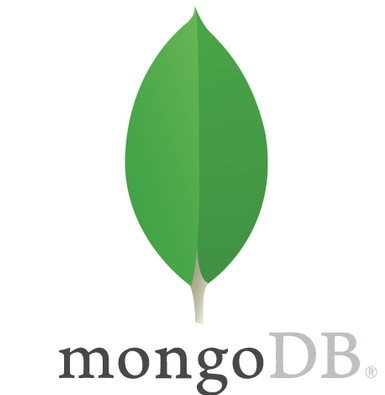
50 important MongoDB interview questions
1. What is MongoDB?
Answer: MongoDB is a popular NoSQL database management system that stores data in flexible, JSON-like documents. It’s known for its scalability, high performance, and flexibility in handling unstructured or semi-structured data.
2. Explain the key features of MongoDB.
Answer: MongoDB offers features like flexible schema design, horizontal scalability through sharding, high availability with replication, support for rich queries, indexing, aggregation framework, and file storage capabilities.
3. What is BSON in MongoDB?
Answer: BSON stands for Binary JSON. It’s the binary-encoded serialization of JSON-like documents used to store data in MongoDB. BSON supports additional data types and is more efficient in representing and accessing data than JSON.
4. Describe the structure of a MongoDB document.
Answer: MongoDB documents are composed of field-value pairs. A document is similar to a JSON object and can contain nested documents or arrays. Each document has a unique _id
field acting as a primary key.
5. What is a MongoDB collection?
Answer: A collection in MongoDB is a group of documents stored in the database. It’s equivalent to tables in relational databases. Collections don’t enforce a schema, allowing for flexibility in data storage.
6. Explain what is Sharding in MongoDB.
Answer: Sharding is a technique used to distribute data across multiple servers. It horizontally partitions data and stores it on different shards, allowing MongoDB to handle large datasets and heavy loads efficiently.
7. What is the role of an Index in MongoDB?
Answer: Indexes in MongoDB improve query performance by allowing faster access to data. They store a small portion of the data set in an easy-to-traverse form. MongoDB uses B-tree indexes by default.
8. Differentiate between MongoDB and RDBMS.
Answer: MongoDB is a NoSQL database, while RDBMS (Relational Database Management System) follows a structured schema. MongoDB uses JSON-like documents, offers flexible schemas, and doesn’t support ACID transactions in the same way as RDBMS.
9. What is the “_id” field in MongoDB?
Answer: The “_id” field is a mandatory field in MongoDB documents. It uniquely identifies each document in a collection. MongoDB automatically adds this field if not explicitly provided.
10. How does MongoDB ensure high availability?
Answer: MongoDB achieves high availability through replication. It creates multiple copies of data across multiple servers (replica set) so that if one server fails, the data remains accessible from other replicas.
11. Explain the aggregation framework in MongoDB.
Answer: The aggregation framework in MongoDB allows users to perform data processing tasks like grouping, filtering, and transforming data using a set of operators. It is used for data aggregation and statistical analysis.
12. What is the role of the WiredTiger storage engine in MongoDB?
Answer: WiredTiger is the default storage engine in MongoDB from version 3.2 onwards. It offers features like compression, document-level concurrency control, and more efficient storage compared to the earlier MMAPv1 storage engine.
13. How does MongoDB handle transactions?
Answer: MongoDB supports multi-document transactions starting from version 4.0. Transactions allow multiple operations to be grouped together, ensuring atomicity, consistency, isolation, and durability (ACID properties).
14. Explain the “find” method in MongoDB.
Answer: The “find” method is used to query data from a MongoDB collection. It retrieves documents that match specified criteria and can be further enhanced with operators like $eq, $gt, $lt, etc., for complex queries.
15. What are some use cases where MongoDB is a good fit?
Answer: MongoDB is suitable for various use cases, including content management systems, real-time analytics, IoT applications, mobile apps, catalog management, and applications requiring a flexible schema.
16. How does MongoDB ensure data consistency in a replica set?
Answer: MongoDB uses the replication protocol called Replica Set Oplog (Oplog) to maintain a log of all write operations. This log is used to replicate changes to all secondary replicas, ensuring data consistency.
17. Explain the role of a “Cursor” in MongoDB.
Answer: A cursor in MongoDB is a pointer to the result set of a query. It’s used to iterate over the query results, fetching documents in batches as needed, reducing memory consumption and improving performance.
18. What is the role of the “mongod” process in MongoDB?
Answer: The “mongod” process is the primary daemon process for the MongoDB server. It manages data requests, performs data storage, retrieval, and other administrative functions.
19. How does indexing improve query performance in MongoDB?
Answer: Indexes in MongoDB help in faster retrieval of data by creating a sorted structure of the indexed fields. They reduce the number of documents that need to be scanned to fulfill a query, thereby improving query performance.
20. Explain the aggregation pipeline in MongoDB.
Answer: The aggregation pipeline in MongoDB is a framework for performing data aggregation operations on documents. It allows data to pass through a sequence of stages, where each stage performs a specific operation on the data, like filtering, grouping, projecting, etc., facilitating complex data transformations.
21. What is GridFS in MongoDB?
Answer: GridFS is a specification used in MongoDB to store and retrieve files that exceed the BSON document size limit (16 MB). It divides a file into smaller chunks and stores each chunk as a separate document, allowing efficient storage and retrieval of large files.
22. Explain the concept of Replication in MongoDB.
Answer: Replication in MongoDB involves creating multiple copies (replicas) of data across different servers. It ensures data redundancy and high availability by maintaining multiple synchronized copies of the data, providing fault tolerance in case of node failure.
23. What is the difference between a replica set and a sharded cluster in MongoDB?
Answer: A replica set in MongoDB consists of multiple nodes where each node contains a copy of the data. It provides redundancy and high availability. In contrast, a sharded cluster is a group of replica sets (shards) used to distribute data across multiple shards for horizontal scaling.
24. How does MongoDB handle schema flexibility?
Answer: MongoDB offers a flexible schema design, allowing documents within a collection to have different structures. Fields can be added, modified, or removed without affecting other documents, providing adaptability to evolving data models.
25. Explain the Write Concern in MongoDB.
Answer: Write Concern in MongoDB determines the acknowledgment level for write operations. It defines the level of acknowledgment required from MongoDB servers regarding successful write operations, ensuring data consistency and durability.
26. What is the role of the “mongos” process in MongoDB?
Answer: The “mongos” process serves as a query router in a sharded cluster setup. It routes client requests to the appropriate shard nodes, facilitating efficient data distribution and retrieval in a sharded environment.
27. Describe the concept of TTL (Time-To-Live) indexes in MongoDB.
Answer: TTL indexes in MongoDB are special indexes used to automatically delete documents after a specified period. They are helpful for managing data that should expire or be purged after a certain time, such as session data or logs.
28. How does MongoDB handle joins between collections?
Answer: MongoDB encourages denormalization by embedding related data within a single document or using references (e.g., ObjectId) between collections. It doesn’t support joins like traditional RDBMS; instead, it promotes data modeling that reduces the need for joins.
29. What is a covered query in MongoDB?
Answer: A covered query is a query in MongoDB where all the fields required by the query are present in an index, and the index itself satisfies the query, eliminating the need to access the actual documents.
30. Explain the concept of the MongoDB Atlas platform.
Answer: MongoDB Atlas is a fully-managed cloud-based database service provided by MongoDB, Inc. It offers a simple and scalable way to deploy, manage, and scale MongoDB databases on popular cloud platforms like AWS, Azure, and Google Cloud.
31. How does MongoDB handle concurrency control?
Answer: MongoDB uses a concurrency control mechanism called Multi-Version Concurrency Control (MVCC) to manage concurrent read and write operations. It allows multiple clients to read data simultaneously while ensuring write operations are isolated.
32. Describe the process of creating indexes in MongoDB.
Answer: Indexes in MongoDB can be created using the createIndex()
method or by specifying indexes in the schema during collection creation. Indexes can be created on single or multiple fields to optimize query performance.
33. What is the role of the Mongoose library in MongoDB?
Answer: Mongoose is an Object-Document Mapper (ODM) library for Node.js and MongoDB. It provides a schema-based solution to model application data, defines schema structures, and provides functionalities for interacting with MongoDB databases using Node.js.
34. Explain the concept of Change Streams in MongoDB.
Answer: Change Streams in MongoDB allow applications to track real-time changes occurring in a database, collection, or cluster. They provide a unified interface for subscribing to and receiving notifications about changes like inserts, updates, or deletions.
35. What are some of the security features provided by MongoDB?
Answer: MongoDB offers various security features, including access control through role-based authentication, Transport Layer Security (TLS/SSL) encryption for data transmission, auditing, authentication mechanisms like LDAP, Kerberos, etc., and field-level redaction.
36. What is the significance of the “ObjectId” in MongoDB?
Answer: The “ObjectId” is a unique identifier generated by MongoDB for each document. It consists of a 12-byte hexadecimal number, containing a timestamp, machine identifier, process ID, and a counter. ObjectId ensures uniqueness within a collection.
37. Explain the differences between MongoDB and Cassandra.
Answer: MongoDB and Cassandra are both NoSQL databases, but they have differences in their data models and architecture. MongoDB uses a flexible schema with JSON-like documents, while Cassandra uses a column-family-based data model with a structured query language (CQL) resembling SQL.
38. What is the role of the “db.stats()” command in MongoDB?
Answer: The “db.stats()” command in MongoDB provides statistics about the current database, such as the size of the data, the number of collections, indexes, and storage utilization, offering insights into database metrics.
39. How does MongoDB handle horizontal scaling?
Answer: MongoDB achieves horizontal scaling through sharding. It distributes data across multiple shards (separate servers or replica sets) based on a shard key. Sharding allows MongoDB to handle large data volumes by partitioning data and balancing the load.
40. Explain the concept of the “Map-Reduce” function in MongoDB.
Answer: Map-Reduce is a data processing technique in MongoDB used for aggregating and processing large volumes of data. It involves two primary functions: “map” to process and map data, and “reduce” to aggregate the mapped data, enabling complex data analysis.
41. What is the role of the “Explain” method in MongoDB?
Answer: The “Explain” method in MongoDB provides information about query execution and performance. It helps analyze query plans, index usage, and execution statistics, assisting in optimizing and fine-tuning query performance.
42. Describe the benefits and limitations of using MongoDB.
Answer: MongoDB offers benefits such as scalability, flexible schema, high availability, horizontal scaling, and support for rich queries. However, it may have limitations in complex transaction handling, joins, and the need for careful data modeling due to its schemaless nature.
43. How does MongoDB handle data consistency in a sharded environment?
Answer: MongoDB ensures data consistency in a sharded environment using the config servers that store metadata related to sharded clusters. The config servers maintain information about the chunk distribution across shards, ensuring consistency during data operations.
44. Explain the concept of a “Geospatial Index” in MongoDB.
Answer: A Geospatial Index in MongoDB is a special index type used for storing and querying geographical data like coordinates (latitude and longitude). It enables efficient querying for location-based data by supporting operations like $near and $geoWithin.
45. What is the purpose of the “Text Index” in MongoDB?
Answer: A Text Index in MongoDB allows for efficient full-text search on string content within documents. It supports text search operations using the $text
operator and is beneficial for searching and ranking textual data.
46. Describe the role of “Geospatial Queries” in MongoDB.
Answer: Geospatial Queries in MongoDB enable operations that involve geographical data. They support queries like finding points within a specified distance from a reference point ($near), searching within defined shapes ($geoWithin), and more.
47. What is the MongoDB Compass tool used for?
Answer: MongoDB Compass is a graphical user interface (GUI) tool designed for interacting with MongoDB databases. It provides a visual representation of data, allowing users to perform CRUD operations, create queries, analyze schemas, and visualize data structures.
48. How does MongoDB handle schema migrations and versioning?
Answer: MongoDB’s flexible schema design reduces the need for explicit schema migrations. However, versioning can be managed by implementing application-level strategies such as embedding version information within documents or using migration scripts during application updates.
49. Explain the role of the “Aggregation Pipeline Optimization” in MongoDB.
Answer: Aggregation Pipeline Optimization in MongoDB involves strategies like query reordering, utilizing indexes, and minimizing data transfer between pipeline stages to optimize the performance of complex data aggregation operations.
50. What is the purpose of the “Partial Indexes” feature in MongoDB?
Answer: Partial Indexes in MongoDB allow creating indexes only on documents that satisfy a specified filter expression. This feature reduces index size and improves performance by indexing a subset of documents matching specific criteria.